Using Azure CLI a lot recently, it has made interactions with Azure so much easier using PowerShell.
https://docs.microsoft.com/en-us/cli/azure/install-azure-cli?view=azure-cli-latest
I had to write a simple PowerShell script that added items to a Storage queue. Once the items were added to a queue an Azure function picked up the items and processed them.
According to the documentation of Azure CLI you need to use az storage message put.
#Login az login #Get the connection string $connectionString = az storage account show-connection-string --name "mystorageaccountname" –resource-group "MyResourceGroup" --query connectionString | ForEach-Object { $PSItem -join '' } | ConvertFrom-Json #Create message $message = "{""Name"":""Hammad"",""LastName"":""Ahmad"",""ID"":""d4b2ffc9-4380-44e4-a0bf-8a9cd58734d2""}" #Add item to the queue az storage message put --content $message --queue-name "myQueueName" --connection-string $connectionString
This fires successfully and I can see the item on the queue. However, when my Azure Function starts to run, I get the error message:
“The input is not a valid Base-64 string as it contains a non-base 64 character, more than two padding characters, or an illegal character among the padding characters.”
To solve this problem you just need to convert the string.
#Login az login #Get the connection string $connectionString = az storage account show-connection-string --name "mystorageaccountname" –resource-group "MyResourceGroup" --query connectionString | ForEach-Object { $PSItem -join '' } | ConvertFrom-Json #Create message $message = "{""Name"":""Hammad"",""LastName"":""Ahmad"",""ID"":""d4b2ffc9-4380-44e4-a0bf-8a9cd58734d2""}" #Encode to bytes $b = [System.Text.Encoding]::UTF8.GetBytes($message) #Convert to Base64String $message64Base = [System.Convert]::ToBase64String($b) #Add item to the queue az storage message put --content $message64Base --queue-name "myQueueName" --connection-string $connectionString
Amazingly the value you push upto the queue is something like below:
“eyJOYW1lIjoiUGF1bCIsIkxhc3ROYW1lIjoiTWF0dGhld3MiLCJJRCI6ImQ0YjJmZmM5LTQzODAtNDZlNC1hMGJmLThhOWNhNTg3MzRkMiJ9”
But it appears on the queue correctly.
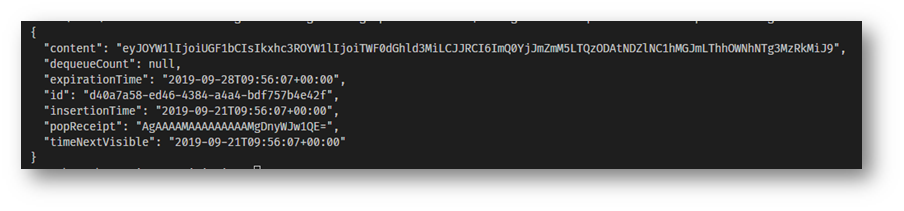
